Key points
- To center the title in ggplot2, use theme(plot.title = element_text(hjust = 0.5))
- To remove the legend in ggplot2, use show.legend = FALSE or legend.position = “none”
- You can customize other aspects of your ggplot2 plots, such as color, size, fill, etc.
- You can create plots with ggplot2, such as histograms, bar charts, line charts, etc.
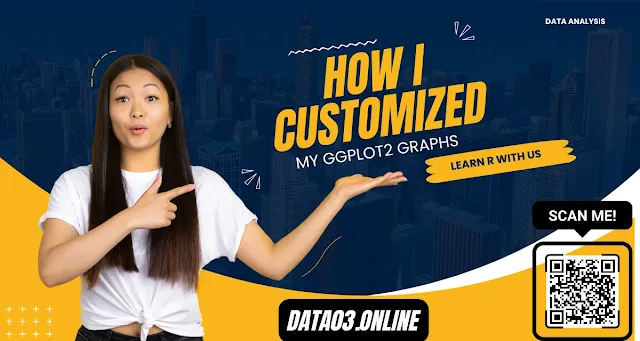
For example, you should center the title of your plot or remove the legend altogether. I'll show you how to do that in this article with simple code. Before Start Make Sure You Have:
- Rstudio: From Installation to Insights
- How to Import and Install Packages in R: A Comprehensive Guide
- Explore Our tutorials of ggplot2
- Say Goodbye to Coding Complex Graphs: Explore the User-Friendly ggplot2-Shiny Combo
Centring the title in ggplot2
theme(plot.title = element_text(hjust = 0.5))
It tells ggplot2 to adjust the plot title's horizontal position (hjust) to 0.5, which means the center. The element_text function allows you to modify other aspects of the text, such as size, colour, font, etc.
Let's see an example of how to center the title in a scatter plot:
Load ggplot2 library
library(ggplot2)
data(mtcars)
Create a scatter plot with the default title
ggplot(mtcars, aes(x = mpg, y = wt)) + geom_point() + ggtitle("Fuel Efficiency vs Weight"))
Center the title
ggplot(mtcars, aes(x = mpg, y = wt)) + geom_point() + ggtitle("Fuel Efficiency vs Weight")+ theme(plot.title = element_text(hjust = 0.5))
Removing the legend in ggplot2
You should remove the legend from your plot, especially if it is unnecessary or takes up too much space.
There are two ways to do that in ggplot2:
- Set show.legend = FALSE in the geom function that creates the legend. This will remove the legend for that specific aesthetic (e.g., color, shape, size, etc.).
- Set legend.position = “none” in the theme function. This will remove all legends from the plot.
Removing the legend in Boxplot
Let’s see an example of how to remove the legend in a box plot:Create a box plot with a default legend
library(ggplot2) ggplot(ToothGrowth, aes(x = dose, y = len)) + geom_boxplot(aes(fill = factor(dose))) + scale_fill_viridis_d() + ggtitle("Tooth Growth by Dose")
Remove the legend for fill
ggplot(ToothGrowth, aes(x = dose, y = len)) + geom_boxplot(aes(fill = factor(dose))) + scale_fill_viridis_d() + ggtitle("Tooth Growth by Dose")+ guides(fill = "none")
Alternatively, you can remove all legends by setting legend.position = “none” in the theme function:
Remove all legends
ggplot(ToothGrowth, aes(x = dose, y = len)) + geom_boxplot(aes(fill = factor(dose))) + scale_fill_viridis_d() + ggtitle("Tooth Growth by Dose")+ theme(legend.position = "none")
Examples of different types of plots
Now that you know how to center the title and remove the legend in ggplot2, let’s see some examples of different types of plots that you can create and customize with ggplot2.Histogram
A histogram is a type of plot showing a numeric variable's distribution. You can use geom_histogram to create a histogram in ggplot2. For example, let’s create a histogram of the mpg variable from the mtcars dataset:
#Load ggplot2 library
library(ggplot2)
#Create a histogram with default settings
ggplot(mtcars, aes(x = mpg)) + geom_histogram() +
ggtitle("Distribution of Miles per Gallon")
#Center the title and remove the legend
ggplot(mtcars, aes(x = mpg)) + geom_histogram() +
ggtitle("Distribution of Miles per Gallon") +
theme(plot.title = element_text(hjust = 0.5), legend.position = "none")
You can also customize other aspects of the histogram, such as the bin width, color, fill, etc. For example:
#Center the title and remove the legend ggplot(mtcars, aes(x = mpg)) + geom_histogram(binwidth = 2, color = "black", fill = "steelblue") + ggtitle("Distribution of Miles per Gallon")+ theme(plot.title = element_text(hjust = 0.5), legend.position = "none")
Bar chart
A bar chart is a type of plot that shows the counts or proportions of a categorical variable. You can use geom_bar to create a bar chart in ggplot2. For example, let’s create a bar chart of the cyl variable from the mtcars dataset:
#Load ggplot2 library library(ggplot2) #Create a bar chart with default settings ggplot(mtcars, aes(x = cyl)) + geom_bar() + ggtitle("Number of Cylinders") #Center the title and remove the legend ggplot(mtcars, aes(x = cyl)) + geom_bar() + ggtitle("Number of Cylinders") + theme(plot.title = element_text(hjust = 0.5), legend.position = "none")
You can also customize other aspects of the bar chart, such as the width, color, fill, etc. For example:
ggplot(mtcars, aes(x = cyl)) + geom_bar(width = 0.8, color = "black", fill = "orange") + ggtitle("Number of Cylinders")
ggplot(mtcars, aes(x = cyl)) + geom_bar(width = 0.8, color = "black", fill = "orange") + ggtitle("Number of Cylinders") + theme(plot.title = element_text(hjust = 0.5), legend.position = "none")
Line chart
A line chart is a type of plot that shows the relationship between two numeric variables, usually over time. You can use geom_line to create a line chart in ggplot2.
For example, let’s create a line chart of the LifeExp by pop from the gapminder dataset:
#Load ggplot2 and gapminder libraries library(ggplot2) library(gapminder) #Create a line chart with default settings ggplot(gapminder, aes(x = lifeExp, y = pop)) + geom_line() + ggtitle("Popullation by LifeExp")
ggplot(gapminder, aes(x = lifeExp, y = pop)) + geom_line() + ggtitle("Popullation by LifeExp") + theme(plot.title = element_text(hjust = 0.5), legend.position = "none")
Create a line chart with custom settings
ggplot(gapminder, aes(x = lifeExp, y = pop)) + geom_line(linewidth = 1, color = "red", linetype = "dashed") + ggtitle("Popullation by LifeExp")
# Center the title and remove the legend ggplot(gapminder, aes(x = lifeExp, y = pop)) + geom_line(linewidth = 1, color = "red", linetype = "dashed") + ggtitle("Popullation by LifeExp") + theme(plot.title = element_text(hjust = 0.5), legend.position = "none")
Conclusion
In this article, you learned how to center the title and remove the legend in your ggplot2 plots. You also saw some examples of different types of plots that you can create and customize with ggplot2. I hope you found this tutorial helpful and informative.
Suppose you want to learn more about data analysis and visualization with R and ggplot2. In that case, you can check out our website, Data Analysis, where we provide tutorials related to RStudio and other topics. Contact us at info@data03.online or hire us for your data analysis projects.
FAQs
What is ggplot2?
ggplot2 is a popular package for creating beautiful and informative plots in R. It is based on the grammar of graphics, which provides a consistent and logical way to describe and build plots.
How do I install ggplot2?
You can install ggplot2 from CRAN using the install.packages function in R:
install.packages("ggplot2")
You can also install the latest development version from GitHub using the devtools package:
devtools::install_github("tidyverse/ggplot2")
How do I load ggplot2?
You can load ggplot2 using the library function in R:
library(ggplot2)
How do I center the title in ggplot2?
You can center the title in ggplot2 using this bit of code:
theme(plot.title = element_text(hjust = 0.5))
How do I remove the legend in ggplot2?
You can remove the legend in ggplot2 using one of these methods:
Set show.legend = FALSE in the geom function that creates the legend.
Set legend.position = "none" in the theme function.
Join Our Community Allow us to Assist You